REST Web Services
A web service is a collection of open protocols and standards used for exchanging data between applications or systems. Software applications written in various programming languages and running on various platforms can use web services to exchange data over computer networks like the Internet in a manner similar to inter-process communication on a single computer. This interoperability (e.g., between Java and Python, or Windows and Linux applications) is due to the use of open standards.
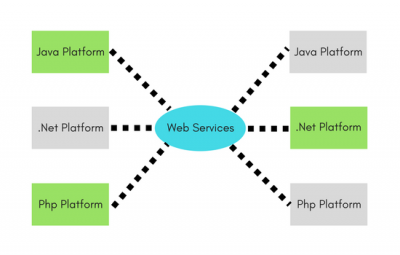
Web services based on REST Architecture are known as RESTful Web Services. These web services use HTTP methods to implement the concept of REST architecture. A RESTful web service usually defines a URI (Uniform Resource Identifier), which is a service that provides resource representation such as JSON and a set of HTTP Methods.RESTFUL web services are lightweight, highly scalable and maintainable and are very commonly used to create APIs for web-based applications.
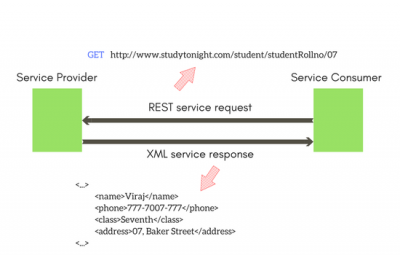
Tools & Technologies used to develop REST Web Service
We will be focusing on developing and deploying REST web services in Java platform, and hence will be using the tools and technologies for the same.
- JAX-RS – Set of annotations and interfaces provided by Java.
- Jersey – Implementation of the JAX-WS.
- Eclipse – Integrated Development Environment (Editor).
- Maven – Generating project structure and build tool.
- Apache Tomcat – Used for deploying the application.
What is JAX-RS?
Java API for RESTFUL Web Services (JAX-RS) is a Java programming language specification that supports creating web services according to the REST architectural pattern in Java. JAX-RS uses annotations, included in Java SE-5, to simplify the deployment of web service clients and the endpoints.
It provides annotations to help in mapping a resource class (a POJO) as a web resource. These annotations include:
- @Path specifies the relative path for a resource class or method.
- @GET, @PUT, @POST, @DELETE specify the HTTP request methods.
- @Produces specify the response type (internet media types), that a particular service will return.
- @Consumes specify the accepted request (internet media types), that a particular
Creating the Resources:
The two important points that must be kept in mind while making the bean class are:
- It should have a default Constructor.
- The bean class is annotated with the annotation @XmlRootElement. This will convert the Java object to XML and vice-versa
As we have made all the member variables as private, therefore create public getter and setter methods for each, to make them accessible.
@XmlRootElement public class Student { private introllNo; private intage; public Student(){} public Student(introllNo, intage) { super(); this.rollNo = rollNo; this.age = age; } public intgetRollNo() { return rollNo; } public void setRollNo(introllNo) { this.rollNo = rollNo; } public intgetAge() { return age; } public void setAge(intage) { this.age = age; }
Now we want to make the other resource class where the server looks for the resource and the corresponding method is called accordingly.
There are a few important things to note here:
- @Path annotation: This annotation will configure the path of the resource.
- @GET annotation: This annotation will configure that which method will be called at the corresponding path. For example: in below case getAllStudents() method will be called when a GET request is made for the /students path.
- @Produces annotation: This annotation will configure in which all formats this method can return the response. In the current example, it can just return application_xml.}
@Path("/students") public class Students { StudentServicestudentService = new StudentService(); @GET @Produces({MediaType.APPLICATION_XML}) public ListgetAllStudents(@Context HttpHeadersheaders) { Liststudents = studentService.getAllStudents(); return students; } }
Creating the Service Class
All the methods of the service class will be called from the resource class methods.
Path Params in GET Request
In JAX-RS, you can use @PathParam annotation to extract the parameter from the request URI and map it to any method. Suppose the client wants the information for resource class. In such a situation, we can use the @PathParam to map this to a method which will return only the requested student’s record.
public class StudentService { public ListgetAllStudents() { List students = new ArrayList(); Students1 = new Student(1, 17); Students2 = new Student(2,18); students.add(s1); students.add(s2); return students; } QaaA }
Now we are left with one more thing, which is the web.xml file in our registration application. The web.xml is the deployment descriptor file, which describes how a component, module or application should be deployed.
For web applications, the deployment descriptor must be web.xml and must reside in WEB-INF directory.
localhost:
The local server host
9812:
This is the port number where the current Apache server (Tomcat) is hosted.
RegistrationApp:
The root path of the app.
rest:
Servlet path is configured to this.
jersey-servlet /rest/*
Path Params in GET Request
In JAX-RS, you can use @PathParam annotation to extract the parameter from the request URI and map it to any method.
Suppose the client wants the information for a student with the roll number 1 or 2 and not for all the students. In such a situation, we can use the @PathParam to map this to a method which will return only the requested student’s record.
@GET @Produces(MediaType.APPLICATION_XM @Path("/{rollno}") public Student getStudentWithRollNo(@PathParam("rollno")introllNo) { Student student = studentService.getStudentWithRollNo(rollNo); return student; }
The @PathParam annotation will map the roll no from the resource URI to the roll No argument of the method.
students:
All the path after the {rest} will be configured in our resources.
Archetype Created Web Application jersey-servlet org.glassfish.jersey.servlet.ServletContainer jersey.config.server.provider.packages com.rest.tutorials.resources 1 jersey-servlet /rest/* Now we are done coding the application and are ready to deploy it on the server
At SPGON our incredibly skilled team of web developers are specialized in developing API’s for web and Mobile applications. We are offering a flexible and cost-effective approach to design and develop cutting-edge API integrations for remote applications. Our team of agile developers will help you to develop API’s for your existing websites and mobile applications. For Effective Rest API’s Please Contact Us or drop us a line at info@spgon.com
Author: Vamsi Krishna Pendurti – Web Developer
Source: studytonight.com